
- //,要在服务器安装office,需要引用using Microsoft.Office.Interop.Word;using Microsoft.Office.Interop.PowerPoint;using Microsoft.Office.Interop.Excel;的dll
- public class FileConvertHelper
- {
- /// <summary>
- /// Ppt转pdf文件
- /// </summary>
- /// <param name="sourcePath"></param>
- /// <param name="targetPath"></param>
- /// <param name="targetFileType"></param>
- /// <returns></returns>
- public static bool PptToPDF(string sourcePath, string targetPath, PpSaveAsFileType targetFileType = PpSaveAsFileType.ppSaveAsPDF)
- {
- bool result;
- object missing = Type.Missing;
- Microsoft.Office.Interop.PowerPoint.ApplicationClass application = null;
- Presentation persentation = null;
- try
- {
- application = new Microsoft.Office.Interop.PowerPoint.ApplicationClass();
- persentation = application.Presentations.Open(sourcePath, MsoTriState.msoTrue, MsoTriState.msoFalse, MsoTriState.msoFalse);
- persentation.SaveAs(targetPath, targetFileType, MsoTriState.msoTrue);
- result = true;
- }
- catch (Exception e)
- {
- string aa = e.Message;
- result = false;
- }
- finally
- {
- if (persentation != null)
- {
- persentation.Close();
- persentation = null;
- }
- if (application != null)
- {
- application.Quit();
- application = null;
- }
- GC.Collect();
- GC.WaitForPendingFinalizers();
- GC.Collect();
- GC.WaitForPendingFinalizers();
- }
- return result;
- }
- /// <summary>
- /// Word转换成pdf
- /// </summary>
- /// <param name="sourcePath">源文件路径</param>
- /// <param name="targetPath">目标文件路径</param>
- /// <returns>true=转换成功</returns>
- public static bool WordToPDF(string sourcePath, string targetPath)
- {
- bool result = false;
- if (File.Exists(targetPath))
- {
- result = true;
- return result;
- }
- string targetDic = Path.GetDirectoryName(targetPath);
- if (!Directory.Exists(targetDic))
- {
- Directory.CreateDirectory(targetDic);
- }
- WdExportFormat exportFormat = WdExportFormat.wdExportFormatPDF;
- object paramMissing = Type.Missing;
- Microsoft.Office.Interop.Word.Application wordApplication = new Microsoft.Office.Interop.Word.Application();
- Document wordDocument = null;
- try
- {
- object paramSourceDocPath = sourcePath;
- string paramExportFilePath = targetPath;
- WdExportFormat paramExportFormat = exportFormat;
- bool paramOpenAfterExport = false;
- WdExportOptimizeFor paramExportOptimizeFor = WdExportOptimizeFor.wdExportOptimizeForPrint;
- WdExportRange paramExportRange = WdExportRange.wdExportAllDocument;
- int paramStartPage = 0;
- int paramEndPage = 0;
- WdExportItem paramExportItem = WdExportItem.wdExportDocumentContent;
- bool paramIncludeDocProps = true;
- bool paramKeepIRM = true;
- WdExportCreateBookmarks paramCreateBookmarks = WdExportCreateBookmarks.wdExportCreateWordBookmarks;
- bool paramDocStructureTags = true;
- bool paramBitmapMissingFonts = true;
- bool paramUseISO19005_1 = false;
- wordDocument = wordApplication.Documents.Open(
- ref paramSourceDocPath, ref paramMissing, ref paramMissing,
- ref paramMissing, ref paramMissing, ref paramMissing,
- ref paramMissing, ref paramMissing, ref paramMissing,
- ref paramMissing, ref paramMissing, ref paramMissing,
- ref paramMissing, ref paramMissing, ref paramMissing,
- ref paramMissing);
- if (wordDocument != null)
- wordDocument.ExportAsFixedFormat(paramExportFilePath,
- paramExportFormat, paramOpenAfterExport,
- paramExportOptimizeFor, paramExportRange, paramStartPage,
- paramEndPage, paramExportItem, paramIncludeDocProps,
- paramKeepIRM, paramCreateBookmarks, paramDocStructureTags,
- paramBitmapMissingFonts, paramUseISO19005_1,
- ref paramMissing);
- result = true;
- }
- catch (Exception ex)
- {
- result = false;
- throw new ApplicationException(ex.Message);
- }
- finally
- {
- if (wordDocument != null)
- {
- wordDocument.Close(ref paramMissing, ref paramMissing, ref paramMissing);
- wordDocument = null;
- }
- if (wordApplication != null)
- {
- wordApplication.Quit(ref paramMissing, ref paramMissing, ref paramMissing);
- wordApplication = null;
- }
- GC.Collect();
- GC.WaitForPendingFinalizers();
- }
- return result;
- }
- /// <summary>
- /// 把Excel文件转换成PDF格式文件
- /// </summary>
- /// <param name="sourcePath">源文件路径</param>
- /// <param name="targetPath">目标文件路径</param>
- /// <returns>true=转换成功</returns>
- public static bool ExcelToPDF(string sourcePath, string targetPath)
- {
- bool result = false;
- if (File.Exists(targetPath))
- {
- result = true;
- return result;
- }
- string targetDic = Path.GetDirectoryName(targetPath);
- if (!Directory.Exists(targetDic))
- {
- Directory.CreateDirectory(targetDic);
- }
- XlFixedFormatType targetType = XlFixedFormatType.xlTypePDF;
- object missing = Type.Missing;
- Microsoft.Office.Interop.Excel.Application application = null;
- Workbook workBook = null;
- try
- {
- application = new Microsoft.Office.Interop.Excel.Application();
- object target = targetPath;
- object type = targetType;
- workBook = application.Workbooks.Open(sourcePath, missing, missing, missing, missing, missing,
- missing, missing, missing, missing, missing, missing, missing, missing, missing);
- workBook.ExportAsFixedFormat(targetType, target, XlFixedFormatQuality.xlQualityStandard, true, false, missing, missing, missing, missing);
- result = true;
- }
- catch (Exception ex)
- {
- result = false;
- throw new ApplicationException(ex.Message);
- }
- finally
- {
- if (workBook != null)
- {
- workBook.Close(true, missing, missing);
- workBook = null;
- }
- if (application != null)
- {
- application.Quit();
- application = null;
- }
- GC.Collect();
- GC.WaitForPendingFinalizers();
- }
- return result;
- }
评价
排名
45
文章
7
粉丝
3
评论
2
基于open office 把各种类型转为pdf在线预览
剑轩 : 都是些高大上的问题!
ICP备案 :渝ICP备18016597号-1
网站信息:2018-2025TNBLOG.NET
技术交流:群号656732739
联系我们:contact@tnblog.net
公网安备:
50010702506256

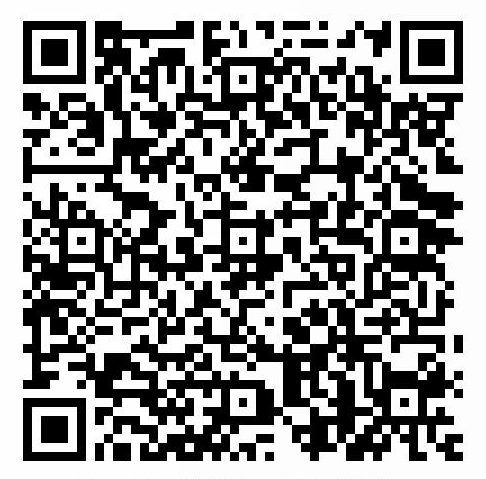
欢迎加群交流技术