
//MVC 实现引进jquery-1.9.1.min.js,highcharts.js
@{
ViewBag.Title = “Index3”;
}
- <script src="~/Content/jquery-1.9.1.min.js"></script>
- <script src="~/Content/highcharts/js/highcharts.js"></script>
- <script type="text/javascript">
- //Highcharts.setOptions({
- // colors: ["#ffabcd", "#abcdff", "#aacc66"]
- //});
- $(function () {
- var parms = {
- chart: {
- type: 'spline'
- },
- title: {
- text: '每日平均温度',
- x: -20 //center
- },
- subtitle: {
- text: '来源: 中国气象局',
- x: -20
- },
- xAxis: {
- categories: ['一月', '二月', '三月', '四月', '五月', '六月',
- '七月', '八月', '九月', '十月', '十一月', '十二月']
- },
- yAxis: {
- title: {
- text: '温度 (°C)'
- },
- plotLines: [{
- value: 0,
- width: 1,
- color: '#808080'
- }]
- },
- tooltip: {
- valueSuffix: '°C'
- },
- legend: {
- layout: 'vertical',
- align: 'right',
- verticalAlign: 'middle',
- borderWidth: 0
- },
- plotOptions: {
- line: {
- dataLabels: {
- enabled: true
- },
- enableMouseTracking: false
- },
- column: {
- dataLabels: {
- enabled: true
- },
- enableMouseTracking: false
- }
- },
- series: []
- };
- //Ajax的get请求获取具体的数据
- $.get('http://localhost:53782/MSChart/GetData', function (data) {
- console.log(data);
- parms.series = data;
- $('#container').highcharts(parms);//把数据填充到容器里,最终输出
- });
- });
- </script>
-
- <div id="container" style="min-width: 310px; height: 400px; margin: 0 auto"></div>
1
//后台实现
数据库设计
create table City
(
Id int primary key identity(1,1),
CityName nvarchar(64)
)
select * from City
create table Wather
(
Id int primary key identity(1,1),
Y decimal(4,2),
X int,
CityId int
)
–drop table Wather
select * from Wather where CityId in (1,2)
insert City values(‘重庆’)
insert City values(‘西永’)
insert City values(‘北京’)
insert Wather values(90,1,1)
insert Wather values(99.5,5,1)
insert Wather values(55.7,9,1)
insert Wather values(15.7,10,1)
insert Wather values(-15.7,11,1)
insert Wather values(67.4,1,1)
insert Wather values(45.5,5,1)
insert Wather values(85.7,3,1)
insert Wather values(95.4,0,1)
insert Wather values(-5.27,2,1)
insert Wather values(99.4,0,1)
insert Wather values(-3.7,3.2,1)
insert Wather values(-50,6,2)
insert Wather values(-9.5,8,2)
insert Wather values(-5.7,10,2)
insert Wather values(-10,6,2)
insert Wather values(-9.5,8,2)
insert Wather values(-2.3,3,2)
insert Wather values(88.5,5,2)
insert Wather values(92.7,9,2)
insert Wather values(92,9,2)
insert Wather values(82.5,5,2)
insert Wather values(45.7,2,2)
insert Wather values(62,3,2)
insert Wather values(20,1,3)
1
insert Wather values(26,2,3)
insert Wather values(-10,3,3)
insert Wather values(40,1,3)
insert Wather values(25,5,3)
insert Wather values(-50,8,3)
insert Wather values(83,1,3)
insert Wather values(56.7,4.3,3)
insert Wather values(-10,9.1,3)
insert Wather values(40,6.4,3)
insert Wather values(89,4.9,3)
insert Wather values(-70,6.7,3)
//视图如下:
//调用数据库
控制器内实现:
- public ActionResult Index3()
- {
- return View();
- }
- public ActionResult GetData()
- {
- //组装好前段需要的数据格式
- List<HighChatModel> highChatModels = new List<HighChatModel>();
- CityDAL cityDAL = new CityDAL();
- WatherDAL watherDAL = new WatherDAL();
- List<City> cities = cityDAL.GetCities();
- //筛选所有需要返回数据的城市id
- List<int?> cityIds = cities.Select(a => (int?)a.Id).ToList();
- List<Wather> needWathers = watherDAL.GetWatherByCityIds(cityIds);
- foreach (City item in cities)
- {
- HighChatModel highChatModel = new HighChatModel();
- highChatModel.name = item.CityName;
- //通过城市id拿到城市对应的温度
- List<Wather> wathers = needWathers.Where(a => a.CityId == item.Id).ToList();
- //组装好数据
- List<decimal?> rwather = new List<decimal?>();
- foreach (Wather wather in wathers)
- {
- rwather.Add(wather.Y);
- }
- highChatModel.data = rwather;
- highChatModels.Add(highChatModel);
- }
- return Json(highChatModels, JsonRequestBehavior.AllowGet);
- }
EF:实体层的应用
- public class WatherDAL
- {
- public List<Wather> GetWather()
- {
- weitherEntities mydbEntities = new weitherEntities();
- List<Wather> cities = mydbEntities.Wather.ToList();
- return cities;
- }
- public List<Wather> GetWatherByCity(int cityId)
- {
- weitherEntities mydbEntities = new weitherEntities();
- List<Wather> cities = mydbEntities.Wather.Where(a => a.CityId == cityId).ToList();
- return cities;
- }
- public List<Wather> GetWatherByCityIds(List<int?> cityIds)
- {
- weitherEntities mydbEntities = new weitherEntities();
- List<Wather> cities = mydbEntities.Wather.Where(a => cityIds.Contains(a.CityId)).ToList();
- return cities;
- }
- }
调用不同类型最终实现效果不同: type: ‘spline’//spline ——— 样条曲线 column ————柱型 pie — 饼 line —线状 area – 区域图
//areaspline — 面积样条曲线 bar — 两边展开 scatter ---- 点型
1.spline ——— 样条曲线
2.column ————柱型
3. pie — 饼状
4.line —线状
5.area – 区域图
6.areaspline — 面积样条曲线
7.bar — 两边展开
8.scatter ---- 点型
//基本就这些了

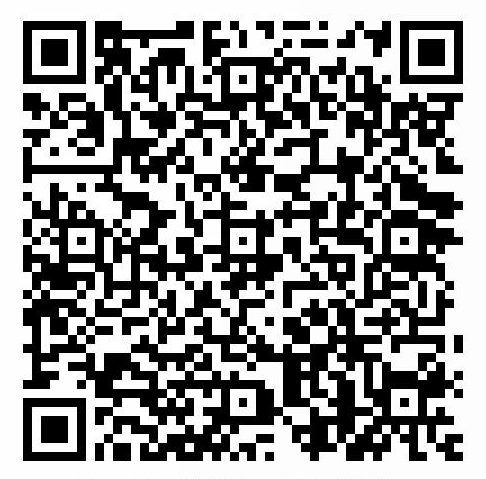